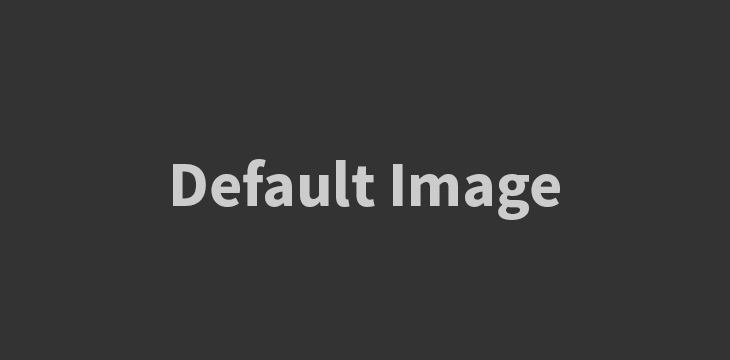
Introduction
In C#, both arrays and ArrayLists are used to store and manipulate collections of data. However, they have some fundamental differences in terms of their implementation, capabilities, and performance. In this article, we will explore the difference between arrays and ArrayLists in C#, their definitions, how they are used, and the advantages and disadvantages of each.
Array: Definition and Usage
An array is a fixed-size collection of elements of the same type. It provides a way to store multiple values of the same data type in contiguous memory locations. Arrays in C# are declared by specifying the data type and the size of the array.
Declaration and Initialization of an Array
To declare and initialize an array in C#, you can use the following syntax: “`csharp dataType[] arrayName = new dataType[size]; “` For example, to create an array of integers with a size of 5, you can use: “`csharp int[] numbers = new int[5]; “`
Accessing Array Elements
Array elements in C# are accessed using zero-based indexing. The index represents the position of an element in the array. To access an element, you use the following syntax: “`csharp arrayName[index] “` For example, to access the first element of the numbers array, you would use: “`csharp int firstNumber = numbers[0]; “`
Advantages and Disadvantages of Arrays
# Advantages:
- – Arrays have a fixed size, which allows for efficient memory allocation and access.
- – Array elements can be accessed directly using indexing, which provides fast and constant-time access.
# Disadvantages:
- – Arrays have a fixed size and cannot be resized dynamically.
- – Inserting or deleting elements in an array requires shifting elements, which can be inefficient.
ArrayList: Definition and Usage
An ArrayList is a dynamic collection that can grow or shrink in size at runtime. It is part of the System.Collections namespace in C#. Unlike arrays, ArrayLists can store elements of different types and automatically handle resizing.
Declaration and Initialization of an ArrayList
To declare and initialize an ArrayList in C#, you can use the following syntax: “`csharp ArrayList arrayListName = new ArrayList(); “`
Adding and Accessing Elements in an ArrayList
To add elements to an ArrayList, you can use the `Add()` method: “`csharp arrayListName.Add(element); “` To access elements in an ArrayList, you can use indexing similar to arrays: “`csharp var element = arrayListName[index]; “`
Advantages and Disadvantages of ArrayLists
# Advantages:
- – ArrayLists can dynamically grow or shrink in size, allowing for flexibility in adding or removing elements.
- – ArrayLists can store elements of different types, providing versatility in handling heterogeneous data.
# Disadvantages:
- – ArrayLists store elements as objects, which can result in performance overhead due to boxing and unboxing operations.
- – ArrayLists do not provide compile-time type safety, which can lead to runtime errors if elements are not properly cast.
Differences Between Array and ArrayList
- 1. Type Safety: Arrays in C# are type-safe, meaning they can only store elements of the declared data type. ArrayLists, on the other hand, can store elements of different types since they store objects.
- 2. Size Flexibility: Arrays have a fixed size defined at the time of declaration and cannot be resized. ArrayLists, on the other hand, can dynamically grow or shrink in size based on the number of elements added or removed.
- 3. Memory Allocation: Arrays allocate contiguous memory blocks for their elements, resulting in efficient memory usage. ArrayLists, however, use an underlying array that is resized as needed, potentially causing memory fragmentation.
- 4. Performance: Arrays provide fast and constant-time access to elements through indexing. ArrayLists, on the other hand, have slower access times due to the need for casting and boxing/unboxing operations.
- 5. Compile-time Safety: Arrays provide compile-time type safety, ensuring that only elements of the specified data type can be stored. ArrayLists do not provide compile-time type safety, which can lead to runtime errors if elements are not properly cast.
Frequently Asked Questions (FAQs)
1. Can an array store elements of different types?
No, arrays in C# can only store elements of the same type. Each array has a specified data type that is determined at the time of declaration.
2. Can an ArrayList be resized to a smaller size?
Yes, an ArrayList in C# can be resized to a smaller size by removing elements using the `Remove()` method or by assigning a new size to the ArrayList using the `Capacity` property.
3. Which one is faster, an array or an ArrayList?
Arrays in C# are generally faster than ArrayLists because they provide direct and constant-time access to elements through indexing. ArrayLists, on the other hand, involve additional operations such as casting and boxingand unboxing, which can impact performance.
4. Can an ArrayList store null values?
Yes, an ArrayList in C# can store null values since it can store elements of different types, including reference types that can have a null value.
5. Can an ArrayList be sorted?
Yes, an ArrayList in C# can be sorted using the `Sort()` method. However, since ArrayLists can store elements of different types, the sorting order may vary based on the data type.
6. Can an array be resized after declaration?
No, arrays in C# have a fixed size that is determined at the time of declaration. To resize an array, a new array with the desired size needs to be created, and the elements from the original array can be copied over.
Conclusion
In conclusion, arrays and ArrayLists are both used to store collections of data in C#, but they have significant differences in terms of their flexibility, performance, and type safety. Arrays provide a fixed-size collection with efficient memory allocation and fast access times. On the other hand, ArrayLists offer dynamic resizing capabilities and the ability to store elements of different types. It is important to consider the specific requirements of your application when choosing between arrays and ArrayLists to ensure optimal performance and functionality.