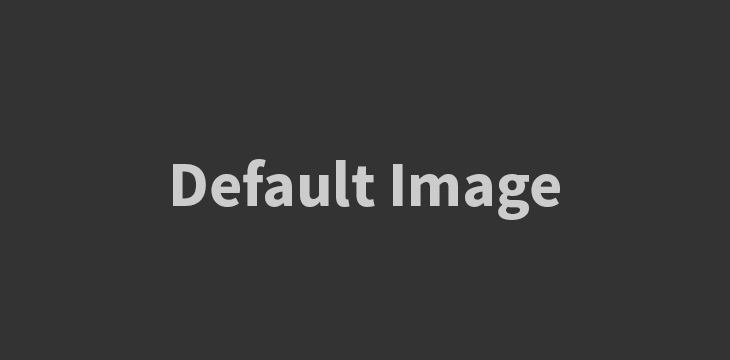
Introduction
In the world of computer science and programming, the efficiency of algorithms plays a crucial role in solving problems. Searching and sorting algorithms are fundamental tools used to organize data and retrieve information quickly and accurately. In this article, we will delve into the intricacies of various searching and sorting algorithms, exploring their principles, strengths, and weaknesses.
Searching Algorithms: Finding the Needle in the Haystack
Linear Search
Linear search is the simplest and most straightforward searching algorithm. It involves sequentially checking each element in a list until the desired element is found or the entire list is traversed. This algorithm is suitable for unordered lists but becomes inefficient for larger datasets.
Binary Search
Binary search is a more efficient searching algorithm that relies on a sorted list. It follows a divide-and-conquer approach by repeatedly dividing the list in half and comparing the target element with the middle element. By discarding the half of the list that does not contain the target, binary search quickly narrows down the search space. This algorithm has a time complexity of O(log n), making it highly efficient for large datasets.
Hashing
Hashing is a searching technique that utilizes a hash function to map keys to specific locations in a data structure called a hash table. By generating a unique hash value for each key, hashing allows for constant-time access to elements, regardless of the size of the dataset. This makes it suitable for scenarios that require fast retrieval of data, such as database management systems.
Sorting Algorithms: Putting Things in Order
Bubble Sort
Bubble sort is a simple and intuitive sorting algorithm that repeatedly compares adjacent elements and swaps them if they are in the wrong order. This process is repeated until the entire list is sorted. Bubble sort has a time complexity of O(n^2) and is not suitable for large datasets due to its inefficiency.
Insertion Sort
Insertion sort is another simple sorting algorithm that builds the final sorted list one element at a time. It iteratively compares each element with the elements before it and inserts it into the correct position. Insertion sort has a time complexity of O(n^2) on average but performs well on small or partially sorted datasets.
Selection Sort
Selection sort divides the list into two parts: a sorted portion and an unsorted portion. It repeatedly finds the minimum element from the unsorted portion and swaps it with the first element of the unsorted portion. This process continues until the entire list is sorted. Selection sort has a time complexity of O(n^2) and is generally less efficient than other sorting algorithms for large datasets.
Merge Sort
Merge sort is a divide-and-conquer sorting algorithm that recursively divides the list into smaller sublists, sorts them, and merges them back together. By repeatedly merging sorted sublists, merge sort achieves a time complexity of O(n log n) and is highly efficient for large datasets. However, it requires additional memory for the merging process.
Quick Sort
Quick sort is another divide-and-conquer algorithm that partitions the list around a chosen pivot element. It repeatedly partitions the list into two sublists, one containing elements smaller than the pivot and the other containing elements larger than the pivot. Quick sort has an average time complexity of O(n log n) but can degrade to O(n^2) in the worst-case scenario. Despite this, it is widely used due to its efficiency and versatility.
Heap Sort
Heap sort utilizes a binary heap data structure to sort elements. It first constructs a max heap (or min heap) from the list and repeatedly extracts the maximum (or minimum) element, swapping it with the last element of the heap. This process continues until the entire list is sorted. Heap sort has a time complexity of O(n log n) and is often used when a stable sort is not required.
Conclusion
Searching and sorting algorithms are indispensable tools in the field of computer science. They allow us to efficiently navigate and organize vast amounts of data, enabling the development of fast and robust applications. Whether it’s finding the desired element in a dataset or arranging elements in a specific order, understanding the principles and characteristics of these algorithms is essential for every programmer. By incorporating these algorithms into our code, we can optimize performance, reduce complexity, and unlock the full potential of our applications. So, the next time you’re faced with a data-related challenge, remember the power of searching and sorting algorithms and choose the one that best suits your needs. Stay in character and keep exploring the fascinating world of algorithms!